22 October 2018
Creating a Javascript spellingchecker with bjspell
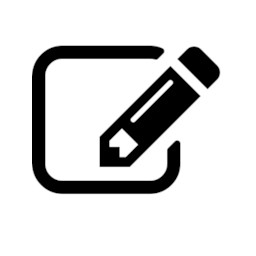
I saw this question on stackoverflow: Need Client side spell checker for DIV. There are not a lot of libraries available that will allow you to do this but I did find an older library called bjspell which does the job.
BJSpell in production: spell-checker.site
Let’s start with writing the HTML. We need a contenteditable div with the spellcheck attribute set to false. This is because we don’t want to use the default browser spellchecking, we want our own implementation.
<div id="text" contenteditable spellcheck="false"></div>
<button id="check" disabled>Check</button>
<button id="reset">Reset</button>
It’s best to add some css rules to the contenteditbale div because we want to style it as an inputfield. The minimum height is required because otherwise the div will collapse to height = 0. Also, let’s add some styling to correct and misspelled words, so users can spot their spelling mistakes.
#text {
min-height: 100px;
border: 1px solid #ccc;
}
#text .correct {
border-bottom: 2px solid green;
}
#text .misspelled {
border-bottom: 2px solid red;
}
Let’s load in bjspell from the bjspell repository. In this example I’m using the en_US dictionary. The available dictionaries are de_DE, en_GB, en_USE, es_ES, fr_FR, it_IT. You can also compile your own but that’s beyond the scope of this tutorial. Call the BJSpell function with url of the dictionary, and a callback. The callback will be fired when bjspell has loaded and parsed the dictionary. In this case, we will set the disabled attriute of the “check button” to false.
var check = document.querySelector('#check');
var div = document.querySelector('#text');use
var reset = document.querySelector('#reset');
var dictionary = 'https://rawcdn.githack.com/maheshmurag/bjspell/master/dictionary.js/en_US.js';
var lang = BJSpell(dictionary, function() {
check.disabled = false;
});
Next, add a click handler to the “check button”. Below is a very simple implementation. You could do a spelling check everytime the div changes, and save/store the user selection and cursor position. In short, we get all words from the div as text. Then we individually check every word for spelling mistakes. If we find a spelling mistake, we add a list of suggestions and save the word as a span element. After we’ve finished mapping every word, we join the all the span elements together and store it in the div’s innerHTML.
check.addEventListener('click', function() {
var text = div.innerText;
var words = text.split(/\s/);
div.innerHTML = words.map(function(word) {
var correct = lang.check(word);
var className = correct ? 'correct' : 'misspelled';
var title = correct
? 'Correct spelling'
: `Did you mean ${lang.suggest(word, 5).join(', ')}?`;
return `<span title="${title}" class="${className}">${word}</span>`;
}).join(' ');
});
We also want a way for our user to reset the div:
reset.addEventListener('click', function() {
div.innerText = div.innerText;
});
Full example:
See the Pen Client-side spellchecking, contenteditable DIV by sempostma (@Afirus) on CodePen.
A complete spellchecker using BJSpell: spell-checker.site
Resources:
InformIT
eBook Store
has a large number of ebooks on a wide range of topics. I would definitely recommend them.
Great courses:
Quickstart
offers a large amount of (online) courses on web development (Use
Code LSOFF50
to get 50% off ;p)
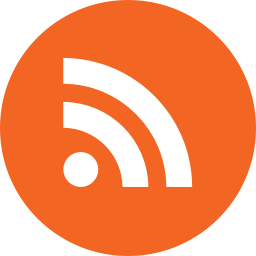
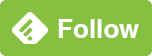